Вот пример радуги для диодов WS2812b. Подключение стандартное. Используем любую ардуину, я использую Arduino Nano.
+5V GND DIN (PIN5)
Отличные переходы. Все цвета. Использовано преобразование HSV -> RGB (смотрите скетч - hsvToRgb).
Для работы с самим диодом есть довольно много библиотек. В моем примере использована AdaFruit NeoPixel. Также пробовал на PololuLedStrip и еще на каких-то.
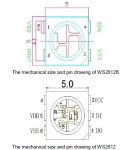
#include <Adafruit_NeoPixel.h>
#include <avr/power.h>
// Which pin on the Arduino is connected to the NeoPixels?
// On a Trinket or Gemma we suggest changing this to 1
#define PIN 5
// How many NeoPixels are attached to the Arduino?
#define NUMPIXELS 1
// When we setup the NeoPixel library, we tell it how many pixels, and which pin to use to send signals.
// Note that for older NeoPixel strips you might need to change the third parameter--see the strandtest
// example for more information on possible values.
Adafruit_NeoPixel pixels = Adafruit_NeoPixel(NUMPIXELS, PIN, NEO_GRB + NEO_KHZ800);
void setup() {
// This is for Trinket 5V 16MHz, you can remove these three lines if you are not using a Trinket
#if defined (__AVR_ATtiny85__)
if (F_CPU == 16000000) clock_prescale_set(clock_div_1);
#endif
// End of trinket special code
pixels.begin(); // This initializes the NeoPixel library.
pixels.setBrightness(255); // Set brightness
}
// Converts a color from HSV to RGB.
// h is hue, as a number between 0 and 360.
// s is the saturation, as a number between 0 and 255.
// v is the value, as a number between 0 and 255.
uint32_t hsvToRgb(uint16_t h, uint8_t s, uint8_t v)
{
uint8_t f = (h % 60) * 255 / 60;
uint8_t p = (255 - s) * (uint16_t)v / 255;
uint8_t q = (255 - f * (uint16_t)s / 255) * (uint16_t)v / 255;
uint8_t t = (255 - (255 - f) * (uint16_t)s / 255) * (uint16_t)v / 255;
uint8_t r = 0, g = 0, b = 0;
switch((h / 60) % 6){
case 0: r = v; g = t; b = p; break;
case 1: r = q; g = v; b = p; break;
case 2: r = p; g = v; b = t; break;
case 3: r = p; g = q; b = v; break;
case 4: r = t; g = p; b = v; break;
case 5: r = v; g = p; b = q; break;
}
return pixels.Color(r, g, b);
}
void loop() {
// Update the colors.
uint16_t time = millis() >> 4;
for(int i=0;i<NUMPIXELS;i++){
byte x = (time >> 2) - (i << 3);
pixels.setPixelColor(i, hsvToRgb((uint32_t)x * 359 / 256, 255, 255));
pixels.show(); // This sends the updated pixel color to the hardware.
}
}