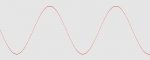
Синусоидальная волна
Частота Частота дискретизации Амплитуда |
void sinus(short amp, float freq, float dfreq, int len, short * outbuf) // амплитуда,частота волны, частота дискретизации,
{ // размер буфера, выходной буфер
float temp = 2.0 * M_PI * freq / dfreq;
float cos = amp;
float sin = 0.0;
outbuf[0] = sin;
for (int i = 1; i < len; i++)
{
sin += temp * cos;
cos -= temp * sin;
outbuf[i] = int(0.5 + sin); // округление
}
}
Пилообразная волна
Частота Частота дискретизации Амплитуда |
void sawtooth(short amp, float freq, float dfreq, int len, short * outbuf)
{
int tempf = int(0.5 + dfreq / freq);
float temp = 2.0 * amp * freq / dfreq;
float t;
int counter = 2;
amp = -amp;
outbuf[0] = amp;
t = amp;
for (i = 1; i < len; i++, counter++)
{
t += temp;
outbuf[i] = t;
if (counter > tempf)
{
outbuf[i] = amp;
t = amp;
counter = 0;
}
}
}
Треугольная волна
Частота Частота дискретизации Амплитуда |
void triangle(short amp, float freq, float dfreq, int len, short * outbuf)
{
int tempf = int(0.5 + dfreq / freq);
int tempf2 = int(0.5 + dfreq/(2 * freq));
float tempa = 4 * amp * freq / dfreq;
int counter = int(0.5 + tempf / 4);
float t;
amp = -amp;
outbuf[0] = 0;
t = 0;
for (i = 1; i < len; i++, counter++)
{
if (counter < tempf2)
{
t += tempa;
outbuf[i] = int(0.5 + t);
}
else
{
if (counter > tempf)
{
counter = 0;
t = 0;
outbuf[i] = amp;
}
else
{
t -= tempa;
outbuf[i] = int(0.5 + t);
}
}
}
}
Прямоугольная волна
Частота Частота дискретизации Амплитуда |
void square(short amp, float freq, float dfreq, int len, short * outbuf)
{
int tempf = int(0.5 + dfreq / freq);
int tempf2 = int (0.5 + dfreq / (freq * 2);
int counter = 2;
outbuf[0] = amp;
for(int i = 1; i < len; i++, counter++)
{
if (counter <= tempf2)
{
outbuf[i] = amp;
}
else
{
outbuf[i] = -amp;
if (counter > tempf)
{
counter = 0;
}
}
}
}
По материалам статьи http://democoder.ru/article/8